About Time and Space Complexity
You did not have much of a chance to analyze the algorithm you used in the
first programming assignment this semester. And the problem you had to solve
was a small one, you had to find the length of the minimum path
between two vertices in a connected,
weighted graph, with only a few vertices.
It's now time to ask yourself, `What if?...' What if I had to solve the
same problem for a graph with ten thousand vertices? What if there were
1 million vertices? How is the running time of my program effected by the
number of vertices in the graph? What about the memory my program requires?
This assignment gives you a chance to find answers to these questions and
to better understand what time and space complexity of an algorithm means.
A description of what you have to do is included in a
memo from your instructor.
Some hints about how to do the experimental part are
also included.
What you'll turn in
- A well commented listing of your program which includes the changes you've done to enable
measuring running time. Grading, as described below, considers both the
functionality and the readability of your code. If you are unsure about
what the documentation of a program means, then you may want to
read
this document.
- A floppy disk (3.5") which contains the source file(s) for your
program, a DOS executable named pa1-time.exe and a README file
indicating what the program does, how to build the executable,
the platform it's been tested on, and how to run it, plus any other information
you consider useful - like the name of the author, etc.
Also include two
input files (use the extension .din for the name), one describing
a graph with 1,000 and another one with 2,000 vertices, and the
spreadsheed (Microsoft Excel) used to record the running times for various
input sizes. You must measure and record the running time of your algorithm for at least 10 graph sizes (N, 2*N, 3*N, 4*N, 5*N, ..., where N is the number of
vertices in the smallest graph.)
- A memo describing your work. You will indicate what you have looked for
and how you solved the problem. Direct the memo to your class instructor.
A hardcopy of the program will be attached to the memo. Also attach tables
with the running times for various input sizes, and the graph(s) that shows
the running time versus the input size.
Grading
- A mark between 0 and 10 for functionality. The mark basically indicates
in what measure your program works properly and how well you have followed
the initial specification.
- A mark between 0 and 10 for readability. This mark indicates how well documented
your program is; it also considers the general appearance of your final
report.
- Multiply the above two marks to get the mark for this programming assignment.
For each business day you turn in your project earlier you receive a 5%
bonus. However, penalties increase by 5% as well. You can turn in your assignment
up to ten business days ahead of the deadline.
Example:
- You turn in your assignment three days earlier: a 3*5=15% bonus will be
added to your assignment mark
- We find a single mistake in the functionality section and the penalty for
that mistake is 0.5 points. Since you have turned the assignment
three days earlier than the deadline, the penalty becomes
0.5*(1+3*5%)=0.5*(1+3*0.05)=0.575
- We calculate the mark by multiplying the marks for the
functionality and readability; in your case the mark for
functionality is 10-0.575=9.425, and the mark for readability is 10.
The result is 9.425*10=94.25
- We add the bonus to this mark: 94.25+94.25*15%=108.38 which we round
to 108. This is your final mark.
You may be asked to do a code review with your instructor.
Hints
- Use the the clock() function that comes with timer.h
- Don't turn in running times of zero! They do not mean we can find
the mininimum length of a path between two vertices in the graph in no time (though we'd like to :)
the fact is caused by the granularity of the computer's timer.
If processing is very fast we may not accumulate at least one CLK_TCK
of time. There are two solutions:
- run the program with an input that's large
enough for the running time to be at least a few CLK_TCKs.
- create a loop that will run the algorithm K times, measure the
time it takes to complete T, then divide T by K to find
how long it took to complete once.
- Keep in mind that the program you run has three major parts:
- read the graph description
- find the length of the minimum path between two vertices
- output the result
Don't include the reading of the graph description and the
print statements in the timing. The reason is that in reality the graph
description comes
from a file (through input redirection), and the output goes into
a file (through output redirection), and reading/writing from/to a file
is a highly system dependent operation. This does not mean that we can
ignore the time it takes to do input/output, it just means it is hard
to get accurate and meaningful readings of these values. And it
may well be the case that the input and output operations actually
determine the running time of your program.
Therefore the time complexity you will determine experimentally, will
be the time complexity of Dijkstra's algorithm, and not the
time complexity for the whole program.
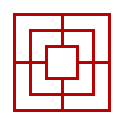 |
Department of
Computer Science
| 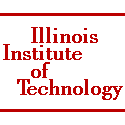 |
DATE: | October 29, 2000 |
TO: | CS-330 students |
FROM: | Your instructor |
SUBJECT: | Space and Time Complexity |
Dear Student,
It just occured to me that the first programming assignment did not call
for any analysis of the algorithm you implemented.
Your program proved to be very successful, to the point that people call
asking about how they can get a hold of your program and whether your program
can handle their problems, some of which are quite large (hundreds and
thousands of vertices in the graph.)
I ask you to analyze the implementation of the algorithm you used in the
first programming assignment and find its time and space complexity. This
is the theoretical part of the problem.
I also ask you to find the time complexity of the algorithm using an
experimental approach, i.e. by measuring the running time of your program
for various input sizes.
All these findings will be included with future releases of your software.
Sincerely yours,
Virgil Bistriceanu
$Id: assign2.html,v 1.1 2002/10/29 14:12:02 virgil Exp $
|